How to Play a Random Sound in Unity C#
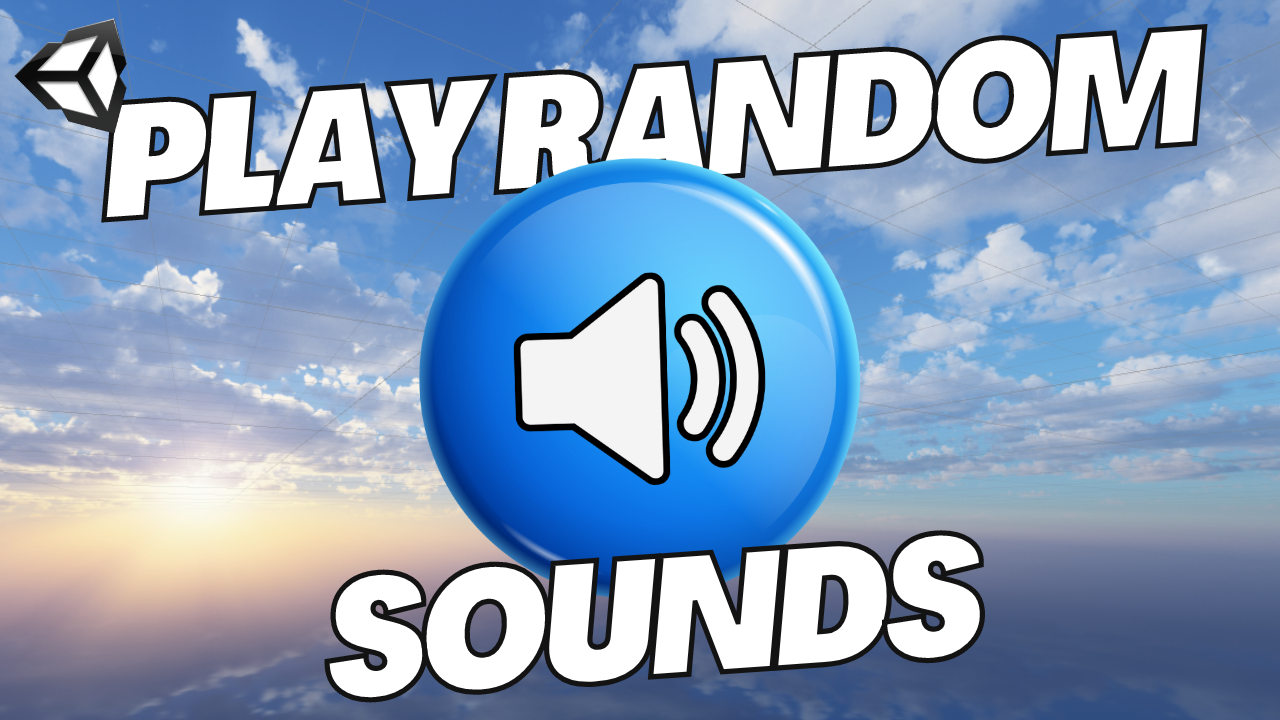
We've all played a game that uses the same sound effect...for everything. It can get exhausting and rip you out of the immersion. What if you wanted to have a list of sound effects that you can randomly play?
Watch the Video:
Setting Up the Environment
Firstly, we need a game object in our scene to house our logic and sounds. Let’s create a new game object and name it Sound Manager
.
The key component required for this setup is an AudioSource. The AudioSource controls what sound gets played. It’s like a record player for a record. You can have a record, you can have 100 records, but without the player, you won’t hear anything. So, ensure that an AudioSource is added to the Sound Manager
object.
Creating the Script
Next, we need to create a script and attach it to our Sound Manager
object. For this example, we’ll name the script PlayRandomSound
.
In the PlayRandomSound
script, we need a reference to the AudioSource we just added. This allows us to add our sound clips and manipulate them as needed. We also need to add the actual sound clips we want to play. Since we want to play multiple sounds, we’ll create a new variable of type AudioClip array.
We also need another variable to hold the actual clip that will be randomly played. Let’s call this variable activeSound
.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayRandomSound : MonoBehaviour
{
public AudioSource audioSource;
public AudioClip[] mySounds;
private AudioClip activeSound;
}
Writing the Logic
With our references set up, we can now write the logic. For simplicity, we’ll trigger the random sound to play when the spacebar is hit.
To set the activeSound
to be a random sound from our array of sounds, we can use the Random.Range
function. This function requires a range, so we’ll specify it to play a sound between index 0 (the start of the array) and the end of the array (the length of the array). This way, whether we have 100 sounds loaded or just 3, the function will account for that.
Finally, we need to call our AudioSource to play one of these random sounds. And that’s it! We’ve set up a system to play a random sound effect in Unity.
void Update()
{
//if I press the spacebar, play a sound
if (Input.GetKeyDown(KeyCode.Space))
{
PlayRandomClip();
}
}
Stay tuned for more Unity tutorials and happy game developing!